catalogs
3, JDBC common interfaces and classes
III. Database creation (MySQL)
(3), get the executor connection
(4), execute the SQL statement, and accept the results
IV. Adding, Deleting, and Re-examining MySQL
1、Get information according to id
I. Beginning
How to Download and Install MySQL First (link (on a website)), how to download and install SQLyog (link (on a website)), and how to download mysql-connector-java.jar (link (on a website)), you can look at the three big guys, here to manipulate the database using the software SQLyog.
Okay, so let’s create the project with idea, and remember to check the box for Web Applications.
Then put mysql-connector-java.jar into the lib inside WEB – INF (if it’s not there, create one yourself, and remember to package the file)
II. Introduction
1, the concept of JDBC
JDBC stands for Java DataBase Connectivity, and is a Java API that can be used to execute SQL statements.It consists of a fewClasses and interfaces written in the java languagecomposition; programmers can make up their own by using thejdbc makes it easy to pass SQL statements to almost any kind of database.。
2、Functions of JDBC
(1) Establish a connection to the database. (2) Send SQL statements to the database and execute them. (3) Processing the results returned by the data.
3, JDBC common interfaces and classes
(1) Driver Interface:Load the driver. (2) DriverManager class:Load the required JDBC driver and programmatically call its methods to create the connection. (3) Connection interface:This class object is used programmatically to create Statement objects. (4)Statement interface:Use this class object to programmatically get the ResultSet object. (5)The ResultSet class:Responsible for storing the query results generated by the execution of the Statement.working schematic
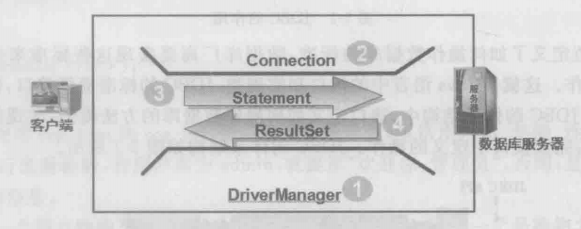
III. Database creation (MySQL)
right click root@localhost establishdb1 (Note: The base character set must be uft8, don’t ask why, I was tortured for half an hour because of this.) Input NameuserOr usesql statementestablish
CREATE DATABASE db1;
USE db1;
CREATE TABLE `user`(
`id` INT,
`name` VARCHAR(30)
);
INSERT INTO `user` VALUES (1, 'Zhang Yi'), (2, 'Zhang Er'), (3, 'Zhang San'), (4, 'Zhang Si'), (5, 'Zhang V').
1. Connecting to MySQL
(1), register the driver
Class.forName("com.mysql.cj.jdbc.Driver");
(2) Getting Connected
Connection com = DriverManager.getConnection("jdbc:mysql://10.16.158.90:3306/db1", "root", "123456");
(3), get the executor connection
Statement stat = com.createStatement();
(4), execute the SQL statement, and accept the results
String sql = "SELECT * FROM user";
ResultSet rs = stat.executeQuery(sql);
(5), processing results
while (rs.next()){
System.out.println(rs.getInt("id") + "\t" + rs.getString("name"));
}
(6) Release of resources
com.close();
stat.close();
com.close();
The code connects.
package com.text.jdbc;
import java.sql.*;
public class jbdc {
public static void main(String[] args) throws Exception {
Class.forName("com.mysql.cj.jdbc.Driver");
Connection com = DriverManager.getConnection("jdbc:mysql://10.16.158.90:3306/db1", "root", "123456");
// DriverManager registers the driver
// Connection database connection object url (specifies the path to the connection Syntax: "jdbc //ip address:port number/database name")
Statement stat = com.createStatement();
// The object that executes the sql statement
String sql = "SELECT * FROM user";
ResultSet rs = stat.executeQuery(sql);
// Execute DML statements with int executeUpdate(Sting sql); int
// Execute DQL statements. ResultSet executeQuery(String sql);
// Object releases void close();
while (rs.next()){
System.out.println(rs.getInt("id") + "\t" + rs.getString("name"));
}
com.close();
stat.close();
com.close();
}
}
Here’s one.little detailIf you forget the name of the driver or make a mistake, you can register the driver in step one.lib -> mysql-connector-java.jar -> jdbc -> Driver![]()
Click Run and the console reads the data from the database and completes the connection.
IV. Adding, Deleting, and Re-examining MySQL
1. Preparation
First we create a database (named db1), a data table (named student), and add the data (this is our summary table), as shown in the figure:
Earlier we used the jdbc classPrint out all data table informationHere we go another way.updated version Here we createcontroller package (which contains the StudentController class), dao package (which contains the StudentDao interface and StudentDaoImpl class), daomian package (which contains the Student class), service package (which contains the StudentService interface and StudentServiceImpl class), StudentServiceImpl class), as shown, and write the code:The StudentController class:
package com.inxzw.domain.controller;
import com.inxzw.domain.domain.Student;
import com.inxzw.domain.service.StudentService;
import com.inxzw.domain.service.StudentServiceImpl;
import org.testng.annotations.Test;
import java.util.ArrayList;
public class StudentController {
private StudentService service = new StudentServiceImpl();
@Test
public void findAll(){
ArrayList<Student> list = service.findAll();
for (Student stu : list){
System.out.println(stu);
}
}
public void findById(){
}
}
StudentDao interface:
package com.inxzw.domain.dao;
import com.inxzw.domain.domain.Student;
import java.util.ArrayList;
public interface StudentDao {
// Look up all student information
public abstract ArrayList<Student> findAll();
//get id
public abstract Student findById(Integer id);
// Add new information
public abstract int insert(Student stu);
// Modify information
public abstract int update(Student stu);
// Delete message
public abstract int delete(Integer id);
}
StudentDaoImpl class:
package com.inxzw.domain.dao;
import com.inxzw.domain.domain.Student;
import java.sql.*;
import java.util.ArrayList;
import java.util.Date;
public class StudentDaoImpl implements StudentDao{
@Override
public ArrayList<Student> findAll(){
Connection com = null;
Statement stat = null;
ResultSet rs = null;
ArrayList<Student> list = new ArrayList<>();
try {
Class.forName("com.mysql.cj.jdbc.Driver");
com = DriverManager.getConnection("jdbc:mysql://10.16.158.90:3306/db1", "root", "123456");
stat = com.createStatement();
String sql = "SELECT * FROM student";
rs = stat.executeQuery(sql);
while (rs.next()){
// System.out.println(rs.getInt("id") + "\t" + rs.getString("name"));
Integer sid = rs.getInt("sid");
String name = rs.getString("name");
Integer age = rs.getInt("age");
Date birthday = rs.getDate("birthday");
Student stu = new Student(sid,name,age,birthday);
list.add(stu);
}
}catch (Exception e){
e.printStackTrace();
}finally {
if (com != null)
{
try {
com.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (stat != null)
{
try {
stat.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (rs != null)
{
try {
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
return list;
}
@Override
public Student findById(Integer id) {
return null;
}
@Override
public int insert(Student stu) {
return 0;
}
@Override
public int update(Student stu) {
return 0;
}
@Override
public int delete(Integer id) {
return 0;
}
}
Student class:
package com.inxzw.domain.domain;
import java.util.Date;
public class Student {
private Integer sid;
private String name;
private Integer age;
private Date birthday;
public Student(Integer sid, String name, Integer age, Date birthday) {
this.sid = sid;
this.name = name;
this.age = age;
this.birthday = birthday;
}
public Integer getSid() {
return sid;
}
public void setSid(Integer sid) {
this.sid = sid;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public Date getBirthday() {
return birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
@Override
public String toString() {// Print
return "Student{" +
"sid=" + sid +
", name='" + name + '\'' +
", age=" + age +
", birthday=" + birthday +
'}';
}
}
StudentService interface:
package com.inxzw.domain.service;
import com.inxzw.domain.domain.Student;
import java.util.ArrayList;
public interface StudentService {
public abstract ArrayList<Student> findAll();
public abstract Student findById(Integer id);
public abstract int insert(String stu);
public abstract int update(Student stu);
public abstract int delete(Integer id);
}
The StudentServiceImpl class:
package com.inxzw.domain.service;
import com.inxzw.domain.dao.StudentDao;
import com.inxzw.domain.dao.StudentDaoImpl;
import com.inxzw.domain.domain.Student;
import java.util.ArrayList;
public class StudentServiceImpl implements StudentService{
private StudentDao dao = new StudentDaoImpl();
public ArrayList<Student> findAll(){
return dao.findAll();
}
public Student findById(Integer id){
return null;
}
@Override
public int insert(String stu) {
return 0;
}
@Override
public int update(Student stu) {
return 0;
}
@Override
public int delete(Integer id) {
return 0;
}
}
In the StudentController class (the controlling class), run findAll(), remember to add @Text, and click Run. Finish searching all information.
V. JDBC Practical Operation
1、Get information according to id
We can get the attribute by id, eg: query the tuple by id of 5. The code is as follows: StudentDaoImpl class:public Student findById(Integer id) {
Student stu = new Student();
Connection com = null;
Statement stat = null;
ResultSet rs = null;
ArrayList<Student> list = new ArrayList<>();
try {
Class.forName("com.mysql.cj.jdbc.Driver");
com = DriverManager.getConnection("jdbc:mysql://10.16.158.90:3306/db1", "root", "123456");
stat = com.createStatement();
String sql = "SELECT * FROM student WHERE sid = '"+id+"' ";
rs = stat.executeQuery(sql);
while (rs.next()){
// System.out.println(rs.getInt("id") + "\t" + rs.getString("name"));
Integer sid = rs.getInt("sid");
String name = rs.getString("name");
Integer age = rs.getInt("age");
Date birthday = rs.getDate("birthday");
stu.setSid(sid);
stu.setName(name);
stu.setAge(age);
stu.setBirthday(birthday);
}
}catch (Exception e){
e.printStackTrace();
}finally {
if (com != null)
{
try {
com.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (stat != null)
{
try {
stat.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (rs != null)
{
try {
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
return stu;
}
The StudentServiceImpl class:
public Student findById(Integer id){
return dao.findById(id);
}
The StudentController class:
public void findById(){
Student stu = service.findById(4); // Query record number 3
System.out.println(stu);
}
Click Run to complete the query.
2. Add information
StudentDaoImpl class: @Override
public int insert(Student stu) {
Connection com = null;
Statement stat = null;
int result = 0;
try {
Class.forName("com.mysql.cj.jdbc.Driver");
com = DriverManager.getConnection("jdbc:mysql://10.16.158.90:3306/db1", "root", "123456");
stat = com.createStatement();
Date d = stu.getBirthday();
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
String birthday = sdf.format(d);
String sql = "INSERT INTO student VALUES ('"+stu.getSid()+"','"+stu.getName()+"','"+stu.getAge()+"','"+birthday+"')";
result = stat.executeUpdate(sql);
}catch (Exception e){
e.printStackTrace();
}finally {
if (com != null)
{
try {
com.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (stat != null)
{
try {
stat.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
return result;
}
The StudentServiceImpl class:
public int insert(Student stu) {
return dao.insert(stu);
}
The StudentController class:
public void insert(){
Student stu = new Student(9, "nine ", 29, new Date());
int result = service.insert(stu);
if (result != 0){
System.out.println("Add Success");
}else {
System.out.println("Failed to add");
}
}
Clicking Run shows that the addition was successful, and we use findAll() to view the table information and complete the addition.
3、Modify the information
StudentDaoImpl class: public int update(Student stu) {
Connection com = null;
Statement stat = null;
int result = 0;
try {
Class.forName("com.mysql.cj.jdbc.Driver");
com = DriverManager.getConnection("jdbc:mysql://10.16.158.90:3306/db1", "root", "123456");
stat = com.createStatement();
Date d = stu.getBirthday();
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
String birthday = sdf.format(d);
String sql = "UPDATE student SET sid = '"+stu.getSid()+"', name = '"+stu.getName()+"', age = '"+stu.getAge()+"',birthday = '"+birthday+"' WHERE sid = '"+stu.getSid()+"'";
result = stat.executeUpdate(sql);
}catch (Exception e){
e.printStackTrace();
}finally {
if (com != null)
{
try {
com.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (stat != null)
{
try {
stat.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
return result;
}
The StudentServiceImpl class:
public int update(Student stu) {
return dao.update(stu);
}
The StudentController class:
@Test
public void update(){
Student stu = service.findById(5);
stu.setName("Zhang 5.5");;
int result = service.update(stu);
if (result != 0){
System.out.println("Modification successful");
}else {
System.out.println("Failed to modify");
}
}
Clicking Run shows that the modification was successful, and we use findAll() to view the table information and complete the modification.
4. Delete information
StudentDaoImpl class:public int delete(Integer id) {
Connection com = null;
Statement stat = null;
int result = 0;
try {
Class.forName("com.mysql.cj.jdbc.Driver");
com = DriverManager.getConnection("jdbc:mysql://10.16.158.90:3306/db1", "root", "123456");
stat = com.createStatement();
String sql = "DELETE FROM student WHERE sid = '"+id+"'";
result = stat.executeUpdate(sql);
}catch (Exception e){
e.printStackTrace();
}finally {
if (com != null)
{
try {
com.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (stat != null)
{
try {
stat.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
return result;
}
The StudentServiceImpl class:
public int delete(Integer id) {
return dao.delete(id);
}
The StudentController class:
@Test
public void delete(){
int result = service.delete(5);
if (result != 0){
System.out.println("Deletion successful");
}else {
System.out.println("Deletion failed");
}
}
Clicking Run shows that the deletion was successful, and we use findAll() to view the table information and complete the deletion (we deleted the information in the row for Zhang 5.5).
VI. Code optimization
Here we have completed the data table to add, delete and check operations, and finally look at theStudentDaoImpl class found that we have written more than 300 lines of code, and we also wrote, very much duplicate code, the efficiency is very bottom, so in order to reduce the amount of code, here we need to optimize.
We create a new package called utils, a new class JDBCutils, and a configuration file config.properties.
Inside the JDBCutils class:”Constructing private methods, declaring required configuration variables, registering drivers, getting database connection methods, releasing resource methods“, the code is as follows:
private JDBCutils(){}
// Declare the required configuration variables
private static String driverClass;
private static String url;
private static String username;
private static String password;
private static Connection con;
// Provide static code blocks, read configuration file information to assign values to variables, register drivers
static {
try {
InputStream is = JDBCutils.class.getClassLoader().getResourceAsStream("config.properties");
Properties prop = new Properties();
prop.load(is);
driverClass = prop.getProperty("driverClass");
url = prop.getProperty("url");
username = prop.getProperty("username");
password = prop.getProperty("password");
} catch (IOException e) {
e.printStackTrace();
}
}
// Get the database connection method
public static Connection getConecction(){
try {
con = DriverManager.getConnection(url,username,password);
} catch (SQLException e) {
e.printStackTrace();
}
return con;
}
//Release of resource methods
public static void close(Connection con, Statement stat, ResultSet rs){
if (con != null){
try {
con.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (stat != null){
try {
stat.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
public static void close(Connection con, Statement stat){
if (con != null){
try {
con.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
config.properties :
driverClass = com.mysql.cj.jdbc.Driver
url = jdbc:mysql://10.16.158.90:3306/db1
username = root
password = 123456
Now that we’ve created our preparations, here’s how findAll() is optimized
First of all, these two steps are interchangeable
Before:
Class.forName("com.mysql.cj.jdbc.Driver");
com = DriverManager.getConnection("jdbc:mysql://10.16.158.90:3306/db1", "root", "123456");
After:
com = JDBCutils.getConecction();
Before:
if (com != null)
{
try {
com.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (stat != null)
{
try {
stat.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (rs != null)
{
try {
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
After:
JDBCutils.close(com, stat, rs);
Doesn’t it feel good to look at it now? Doesn’t it save a lot of code? Click run and see if it runs.Then.The findById(), insert(), update(), delete() methods all work the same way., which is not shown here.OK, it runs without problems and the optimization was successful.
JSP Connection to MYSQL
First, let’s go back to the student table and run theadd, delete and checkOperation.
1、Query operation
We want to query all the information, in fact, the method is similar, and the above MYSQL of the 5 steps are the same, so here will not be one again to explain, directly look at the code.<%@ page language="java" import="java.sql.Connection" contentType="text/html; charset=UTF-8"
pageEncoding="GB18030"%>
<%@ page import="java.sql.*" %>
<%@ page import="java.util.Date" %>
<!DOCTYPE html>
<html>
<head>
<title>JSP Read Database</title>
</head>
<body>
<table border="1" align="center">
<%
String driverClass="com.mysql.jdbc.Driver";
String url="jdbc:mysql://localhost/db1?useUnicode=true&characterEncoding=utf-8&useSSL=false&serverTimezone=UTC";
String user="root";
String password="123456";
Connection conn;
try{
Class.forName(driverClass);
conn=DriverManager.getConnection(url, user, password);
Statement stmt = conn.createStatement();
// Search for all information
String sql = "select * from student";
ResultSet rs = stmt.executeQuery(sql);
while(rs.next()){
Integer id = rs.getInt("id");
String name = rs.getString("name");
Integer age = rs.getInt("age");
Date birthday = rs.getDate("birthday");
out.println(id + " " + name + " " + age + " " + birthday + "<br>");
}
}catch (Exception ex){
ex.printStackTrace();
}
%>
</table>
</body>
</html>
Caution:Here’s a little detail.In mysql version 6.0, you must configure the operation! In the properties configuration file, it is not correct to write the url address alone, you need to add the url text at the end of the text. ?useUnicode=true&characterEncoding=utf-8&useSSL=false&serverTimezone=UTC This sentence. Be sure to add.I Debug for more than an hour, don’t be fooled by the java syntax, make sure you add it!!!!
2. Increase operation
Let’s look at the code first<%@ page language="java" import="java.sql.Connection" contentType="text/html; charset=UTF-8"
pageEncoding="GB18030"%>
<%@ page import="java.sql.*" %>
<%@ page import="java.util.Date" %>
<!DOCTYPE html>
<html>
<head>
<title>JSP Read Database</title>
</head>
<body>
<table border="1" align="center">
<%
String driverClass="com.mysql.jdbc.Driver";
String url="jdbc:mysql://localhost/db1?useUnicode=true&characterEncoding=utf-8&useSSL=false&serverTimezone=UTC";
String user="root";
String password="123456";
Connection conn;
try{
Class.forName(driverClass);
conn=DriverManager.getConnection(url, user, password);
Statement stmt = conn.createStatement();
String sql = "insert into student(id,name,age,birthday) VALUES (10,' sheet ten ',23,'2000-12-23')";
int rs = stmt.executeUpdate(sql);
if(rs == 0 ){
out.print("Failed to increase");
}else {
out.print("Increase successful");
}
}catch (Exception ex){
ex.printStackTrace();
}
%>
</table>
</body>
</html>
attention (heed):Here’s another detail (details are hair for hair, woo hoo hoo), after our server startup is complete, here must be executed in the browser, otherwise, there is no operation on the database.Definitely something to remember!!!Refresh the database to see the effect
3、Modify the operation
Here we want to change the id of “Zhang X” to 12, so the corresponding statement isUPDATE student SET id = 12 where name=’ ten ‘Let’s look at the code.<%@ page language="java" import="java.sql.Connection" contentType="text/html; charset=UTF-8"
pageEncoding="GB18030"%>
<%@ page import="java.sql.*" %>
<%@ page import="java.util.Date" %>
<!DOCTYPE html>
<html>
<head>
<title>JSP Read Database</title>
</head>
<body>
<table border="1" align="center">
<%
String driverClass="com.mysql.jdbc.Driver";
String url="jdbc:mysql://localhost/db1?useUnicode=true&characterEncoding=utf-8&useSSL=false&serverTimezone=UTC";
String user="root";
String password="123456";
Connection conn;
try{
Class.forName(driverClass);
conn=DriverManager.getConnection(url, user, password);
Statement stmt = conn.createStatement();
String sql="UPDATE student SET id = 12 where name=' ten '";
int rs = stmt.executeUpdate(sql);
if(rs == 0 ){
out.print("Failed to modify");
}else {
out.print("Modification successful");
}
}catch (Exception ex){
ex.printStackTrace();
}
%>
</table>
</body>
</html>
Click Run, remember to run it once in the browser, ok, complete the modificationThe effect is as follows
4、Delete operation
Let’s remove the condition where id is 12, here again we first give the statement “DELETE FROM student WHERE id=12″ Let’s look at the code first<%@ page language="java" import="java.sql.Connection" contentType="text/html; charset=UTF-8"
pageEncoding="GB18030"%>
<%@ page import="java.sql.*" %>
<%@ page import="java.util.Date" %>
<!DOCTYPE html>
<html>
<head>
<title>JSP Read Database</title>
</head>
<body>
<table border="1" align="center">
<%
String driverClass="com.mysql.jdbc.Driver";
String url="jdbc:mysql://localhost/db1?useUnicode=true&characterEncoding=utf-8&useSSL=false&serverTimezone=UTC";
String user="root";
String password="123456";
Connection conn;
try{
Class.forName(driverClass);
conn=DriverManager.getConnection(url, user, password);
Statement stmt = conn.createStatement();
String sql="DELETE FROM student WHERE id=12";
int rs = stmt.executeUpdate(sql);
if(rs == 0 ){
out.print("Failed to delete");
}else {
out.print("Deletion successful");
}
}catch (Exception ex){
ex.printStackTrace();
}
%>
</table>
</body>
</html>
Here the browser shows that the deletion was successfulRefresh to see the effect
wrap-up: everyone in theStudentDaoImpl There is a small detail in the classDriverManager.getConnection set (“jdbc //ip address:port number/database name”) ip address here, in a different place to write the code, must remember to change, I debugged an hour before I found that I have been wondering why the morning and afternoon in the I’ve been wondering why it works in the library in the morning and afternoon, but not in the dormitory at night. I’ve been wondering why it works in the library in the morning and afternoon, but not in the dormitory at night. It’s a bloody pain in the ass!